For all the developers who have been searching for a robust platform for building mobile and web application faster, you can think about Firebase. Firebase is a Backend-as-a-Service — BaaS — that started and grew up into a next-generation app-development platform on Google Cloud Platform. Implementation of the authentication system with Firebase in web and mobile apps is very easy.
Today, I am going to create a sample application to show the Firebase email and password authentication with Angular 8 Application in which user will be able to login with email and password and register with email and password.
If you don't have an understanding about the connection of Angular applicaion, Read the article my previous article on Firebase How to connect Firebase with Angular 8 Application from scratch
Let' Get Started
Step 1: Create Angular application using angular CLI
Run below command to create Angular 8 application
ng new firebase-auth-angular-app
Now type cd firebase-auth-angular-app on the terminal to move into project folder.
Step 2: Create Service file to Configure Firebase Authentication
Now, I am going to create a service file that will have logic for enabling sign in, sign up or sign out a user from the Angular 8 Firebase authentication app.
Run below command in Angular CLI to generate authentication service file without spec.ts
file:
ng g s services/Authentication --spec=false
In this file we will have a complete firebase authentication task using AngularFireAuth needed for our application.
import { Injectable } from '@angular/core';
import { AngularFireAuth } from "@angular/fire/auth";
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class AuthenticationService {
userData: Observable<firebase.User>;
constructor(private angularFireAuth: AngularFireAuth) {
this.userData = angularFireAuth.authState;
}
/* Sign up */
SignUp(email: string, password: string) {
this.angularFireAuth
.auth
.createUserWithEmailAndPassword(email, password)
.then(res => {
console.log('You are Successfully signed up!', res);
})
.catch(error => {
console.log('Something is wrong:', error.message);
});
}
/* Sign in */
SignIn(email: string, password: string) {
this.angularFireAuth
.auth
.signInWithEmailAndPassword(email, password)
.then(res => {
console.log('You are Successfully logged in!');
})
.catch(err => {
console.log('Something is wrong:',err.message);
});
}
/* Sign out */
SignOut() {
this.angularFireAuth
.auth
.signOut();
}
}
Step 3: Implement Firebase Authentication Login, Sign up and Sign out in Component
After creating service, We will use that in the component. We will import the auth service in component and create the signup, login and sign out functionality. Now add the below code in app.component.ts file inside app folder.
import { Component } from '@angular/core';
import { AuthenticationService } from './services/authentication.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(
private authenticationService:AuthenticationService
){
}
email: string;
password: string;
signUp() {
this.authenticationService.SignUp(this.email, this.password);
this.email = '';
this.password = '';
}
signIn() {
this.authenticationService.SignIn(this.email, this.password);
this.email = '';
this.password = '';
}
signOut() {
this.authenticationService.SignOut();
}
}
In the above file, at the top, I have injected the Angular Firebase authentication service. Then I declare the authentication methods to consume the firebase authentication methods.
Now at the end, Need to update the component template. Put the below code in app.component.html file
<div class="container">
<div class="row">
<div class="col-md-6 col-md-offset-3">
<h1 *ngIf="authenticationService && authenticationService.userData | async">Hello {{ (authenticationService.userData | async)?.email }}</h1>
<div *ngIf="!(authenticationService.userData | async)">
<div class="form-group">
<label for="email">Email address:</label>
<input type="text" class="form-control" [(ngModel)]="email" placeholder="email">
</div>
<div class="form-group">
<label for="pwd">Password:</label>
<input type="password" class="form-control" [(ngModel)]="password" id="pwd" placeholder="password">
</div>
<button (click)="signUp()" class="btn btn-default">Sign Up</button>
<button (click)="signIn()" class="btn btn-default" style="margin-left:5px;">Login</button>
</div>
<button (click)="signOut()" class="btn btn-primary" *ngIf="authenticationService.userData | async">Logout</button>
</div>
</div>
In the above file, the interface is there to log in and registration of the user. async pipe has been used above to determine the logged-in state of the user.
Step 4: Run the app
Finally, all the needed steps are done, Now run the angular app with npm start and the app will look like below on browser.
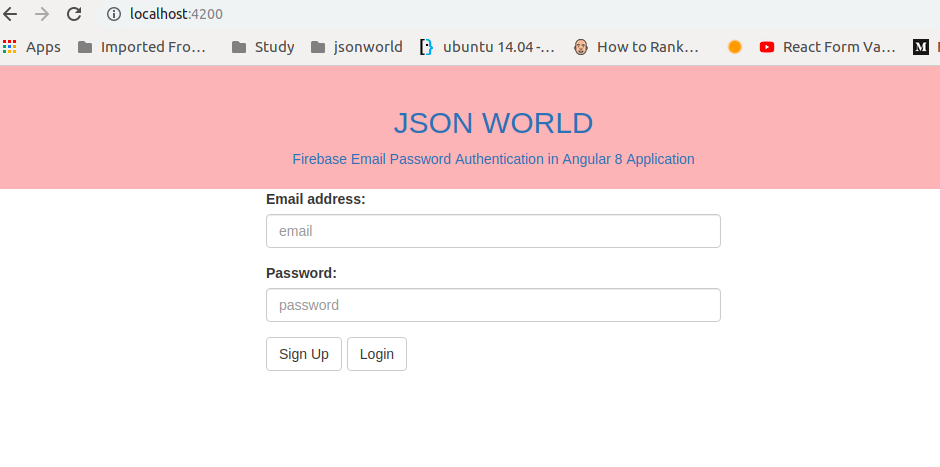
Conclusion
We have successfully emplimented firebase email and password authentication very easily in few minutes only.
If you are new to Angular 8 then find Angular Sample Projects to start the app for enterprise-level application
Let me know your thoughts over the email demo.jsonworld@gmail.com. I would love to hear them and If you like this article, share with your friends.
Thank You!