In this article, We will learn how to work with Radio button in Reactjs app. Working with radio button is little different than other input elements of HTML.
So, here I am going to create a sample application where a basic React component will be used to show the use of radio button in the React app.
Let's Get Started
Step 1: Create React App
create a basic react app using create-react-app tool
npx create-react-app radio-button-app
Once the app is created using above command, Move to project folder using cd radio-button-app/ and start the app using npm start command.
Step 2: Define default Radio Button State
constructor() {
super();
this.state = {
country: ''
};
}
In the above code, We have set the radio button state. Which will be updated as per the user selection.
Step 3: Create Form with Buttons
render() {
return (
<div className="App">
<form onSubmit={this.onSubmit}>
<strong>Select country:</strong>
<ul>
<li>
<label>
<input type="radio" value="india" checked={this.state.country === "india"} onChange={this.onRadioChange} />
<span>India</span>
</label>
</li>
<li>
<label>
<input type="radio" value="australia" checked={this.state.country === "australia"} onChange={this.onRadioChange} />
<span>Australia</span>
</label>
</li>
<li>
<label>
<input type="radio" value="england" checked={this.state.country === "england"} onChange={this.onRadioChange} />
<span>England</span>
</label>
</li>
<li>
<label>
<input type="radio" value="spain" checked={this.state.country === "spain"} onChange={this.onRadioChange} />
<span>Spain</span>
</label>
</li>
</ul>
<button type="submit">Choose Country</button>
</form>
</div>
);
}
In the above form, we have defined 4 radio buttons containing the name of 4 different countries. And with the radio button, we have added two properties checked and onChange. Checked properties in the form actually manage the user selection and update the value to the country state.
Now when the user checks any of the radio buttons then the state is updated using the country variable using the onChange event handler.
Step 4: Complete code
import React, { Component } from 'react';
class App extends Component {
constructor() {
super();
this.state = {
country: ''
};
this.onRadioChange = this.onRadioChange.bind(this);
this.onSubmit = this.onSubmit.bind(this);
}
onRadioChange = (e) => {
this.setState({
country: e.target.value
});
}
onSubmit = (e) => {
e.preventDefault();
console.log(this.state);
}
render() {
return (
<div className="App">
<form onSubmit={this.onSubmit}>
<strong>Select country:</strong>
<ul>
<li>
<label>
<input type="radio" value="india" checked={this.state.country === "india"} onChange={this.onRadioChange} />
<span>India</span>
</label>
</li>
<li>
<label>
<input type="radio" value="australia" checked={this.state.country === "australia"} onChange={this.onRadioChange} />
<span>Australia</span>
</label>
</li>
<li>
<label>
<input type="radio" value="england" checked={this.state.country === "england"} onChange={this.onRadioChange} />
<span>England</span>
</label>
</li>
<li>
<label>
<input type="radio" value="spain" checked={this.state.country === "spain"} onChange={this.onRadioChange} />
<span>Spain</span>
</label>
</li>
</ul>
<button type="submit">Choose Country</button>
</form>
</div>
);
}
In the above code, we can the onSubmit event handler which will trigger on the submit of the form. Have usede.preventDefault() method to prevent the page redirection after form submit.
Working will app looks like below:
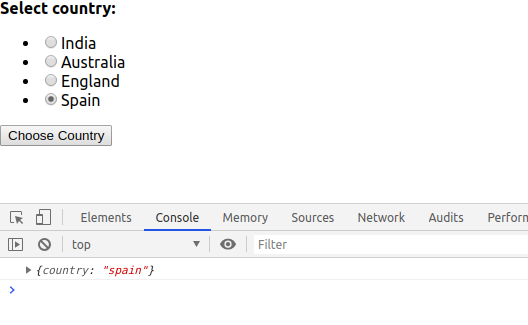
Conclusion
We have successfully created a sample application to work with radio button in react.js app very easily in few minutes only.
If you are new to React then find here Reactjs Sample Projects to start the app for enterprise-level application
Let me know your thoughts over the email demo.jsonworld@gmail.com. I would love to hear them and If you like this article, share it with your friends.
Thank You!