React.js is one of the most popular Javascript frontend framework developed by Facebook. The library was created by the Facebook team as a tool to provide high-performance UI solutions. At its core lies an extraordinary page rendering mechanism, which allows all the content to refresh immediately in response to user actions. The popularity of this framework and demand in the IT industry is also increasing day by day. There are many very popular platforms using React.js for their websites like Netflix, Reddit, Airbnb, Dropbox and many more.
In this tutorial, We will create a React Application to understand its basic flow with a basic sample application to create User Registration and Login Application using Node.js & Express.js at the server end.
Below are the requirements for creating the CRUD on MEAN
- Node.js
- React
- MongoDB
- IDE or Text Editor
I assume that you have already available the above tools/frameworks and you are familiar with all the above that what individually actually does. Now have a look on the basic folder structure for the app.
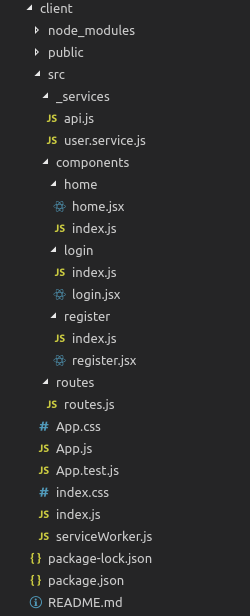
In the above folder structure, _services is responsible for making an HTTP request in the application, the components folder is containing the components needed in our basic app, routes folder is having all the routes defined in the application.
Now we will proceed step by step to accomplish the task.
Create a New Project
Create a new project with the below command:
create-react-app user-registration-login-app
If you have yarn package manager installed proceed accordingly.
Open localhost:3000 in your browser to see the basic react app in running.
Now we will install Axios using NPM for sending the HTTP request at backend to fetch data from server.
Now we will create the needed components Register, Login, Home
Register component
Have a look for code inside register.jsx file(components/register/register.jsx)
import React from 'react';
import {Link, withRouter } from 'react-router-dom'
import ApiFun from '../../_services/api'
class Register extends React.Component{
constructor(props){
super(props);
this.state={
firstName:'',
lastName:'',
username:'',
email:'',
password:''
}
}
getRegister=()=>{
ApiFun.postApi('register',{firstName:this.state.firstName,lastName:this.state.lastName,userName:this.state.username,email:this.state.email,password:this.state.password})
.then(json => {
console.log(json.data,'response of register api----');
if(json.data.responseCode===200){
this.props.history.push('/login')
}
else{
this.props.history.push('/register')
}
}).catch((error)=>{
console.log("error-----------",error)
})
// this.props.history.push('/login')
}
handleChange= (e)=> {
this.setState({[e.target.name]:e.target.value});
}
render(){
return (
<div className="col-md-6 col-md-offset-3">
<h2>Registration Form</h2><form>
<div className='form-group'>
<label>First Name:</label>
<input type="text" name="firstName" className="form-control" onChange={this.handleChange} value={this.state.firstName} />
</div>
<div className='form-group'>
<label>Last Name:</label>
<input type="text" name="lastName" className="form-control" onChange={this.handleChange} value={this.state.lastName} />
</div>
<div className='form-group'>
<label>Username:</label>
<input type="username" name="username" className="form-control" onChange={this.handleChange} value={this.state.username} />
</div>
<div className='form-group'>
<label>Email:</label>
<input type="email" name="email" className="form-control" onChange={this.handleChange} value={this.state.email} />
</div>
<div className='form-group'>
<label>Password: </label>
<input type="password" name="password" className="form-control" onChange={this.handleChange} value={this.state.password} />
</div>
<button type="button" className="btn btn-primary" onClick={this.getRegister}>Register</button>
<Link to="/login" className="btn btn-link">Login</Link>
</form></div>
)
}
}
export default withRouter(Register);
In the above file(Register Component) renders a simple registration form with fields, firstName, lastName, username, email, password, and a button to submit the form. The available fields are also defined in state and updating on change of values in it with the help of handleChange function. On submit of the form getRegister() method is called which is defined at the top.
There is also index.js file in register folder containing basic export code:
import Register from './register'
export default Register
Login Component
Have a look for code inside login.jsx file
import React from 'react';
import { Link, withRouter } from 'react-router-dom'
import ApiFun from '../../_services/api'
class Login extends React.Component{
constructor(props){
super(props)
this.state = {
email:'',
password:'',
goto: false
}
}
componentWillMount(){
console.log("Component will mount")
}
getLogin=()=>{
console.log(`in login`,this.state.email,this.state.password)
ApiFun.postApi('login',{email:this.state.email,password:this.state.password})
.then(json => {
if(json.data.result && json.data.result.length>0){
this.props.history.push('/home')
}
else{
this.props.history.push('/login')
}
}).catch((error)=>{
alert('login failed. Try later!')
})
}
handleChange= (e)=> {
this.setState({[e.target.name]:e.target.value});
}
render(){
return (
<div className="col-md-6 col-md-offset-3">
<h2>Login Form</h2>
<form name="form">
<div className='form-group'>
<label>Email:</label>
<input type="email" name="email" className="form-control" onChange={this.handleChange} value={this.state.email} />
</div>
<div className='form-group'>
<label>Password: </label>
<input type="password" name="password" className="form-control" onChange={this.handleChange} value={this.state.password} />
</div>
<button type="button" className="btn btn-primary" onClick={this.getLogin}>Login</button>
<Link to="/register" className="btn btn-link">Register</Link>
</form>
</div>
)
}
}
export default withRouter(Login);
In the above file(Login Component) renders a simple login form with fields, email, password, and a button to submit the form. The available fields are also defined in state and updating on change of values in it with the help of handleChange function. On submit of the form getLogin() method is called which is defined at the top.
Home Component
import React from 'react';
class Home extends React.Component{
getHome=()=>{
console.log(`in home`)
}
logout =() =>{
this.props.history.push('/login')
}
render(){
return <div>
<h2>Login Successful!</h2>
<h3>Welcome to Home Page</h3>
<button className="btn btn-primary" onClick={this.getHome}>Home</button>
<button className="btn btn-default" onClick={this.logout}>Logout</button>
</div>
}
}
export default Home;
In the above file(Home Component) just showed the login success message.
Services
Have a look in code inside api.js(_services/api.js)
// import React from 'react';
import axios from 'axios'
const baseUrl="http://localhost:3001/auth/"
class ApiFun {
static postApi(url,data){
return axios.post(baseUrl + url, data);
}
static getApi(url){
return axios.get(baseUrl + url);
}
}
export default ApiFun
Now we will see the code inside user.service.js
import React from 'react';
import axios from 'axios'
const baseUrl="http://localhost:3001/"
class UserService {
static register(url,data){
return axios.post(baseUrl + url, data);
}
static getApi(url){
return axios.get(baseUrl + url);
}
}
export default UserService
Now the next part is routes.js(routes/routes.js)
import Login from '../components/login/index';
import Register from '../components/register/index';
import Home from '../components/home/index';
export const routes = [
{
path: '/',
exact: true,
name: 'Login',
component: Login
},
{
path: '/login',
// exact: true,
name: 'Login',
component: Login
},
{
path: '/register',
name: 'Register',
component: Register
},
{
path: '/home',
name: 'Home',
component: Home
},
]
Needed routes have been defined in the above file.
Now the last file we need to update is App.js in src folder
import React, { Component } from 'react';
// import logo from './logo.svg';
import { BrowserRouter as Router, Route, Switch} from "react-router-dom";
import './App.css';
import Login from './components/login/index'
import Register from './components/register/index'
import { routes } from '../src/routes/routes'
class App extends Component {
render() {
return (
<div className="jumbotron">
<div className="container">
<div className="col-sm-8 col-sm-offset-2">
<Router>
<Switch>
<Route exact path="/" component={Register} />
<Route path="/login" component={Login} />
<Route path="/register" component={Register} />
{
routes.map(r=><Route key={r.path} path={r.path} exact={r.exact} component={r.component} name={r.name} />)
}
</Switch>
</Router>
</div>
</div>
</div>
);
}
}
export default App;
Server End Task:
Please find the detailed blog on creating a server for user registration and login application Start Creating APIs in Node.js with Async/Await
Conclusion
In this demo, we understand the Basic User Registration and login in Reactjs using Node.js & Express.js at the server end. You can find other demos at Reactjs Sample Projects
That’s all for now. Thank you for reading and I hope this post will be very helpful for Basic React User Registration Login App Without Redux.
Let me know your thoughts over email demo.jsonworld@gmail.com. I would love to hear them and If you like this article, share it with your friends.
Thank You!