In this article, I am going to explain about Select dropdown in Angular reactive forms.
Let's Get Started
Step 1: Create New Angular app
At first create a new angular app with below command on terminal/command promp.
ng new angular-select-dropdown
Step 2: Inject Reactive Form within the app
import { ReactiveFormsModule } from '@angular/forms';
@NgModule({
imports: [
ReactiveFormsModule
]
})
Step 3: Update app.component.ts file
In this file, we will write all the logical task needed to do, like creation of dummy country list, form initialization, getter method for accessing form control etc. update app.component.ts file with below code.
import { Component } from '@angular/core';
import { FormBuilder, Validators } from "@angular/forms";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
isSubmitted = false;
// Country Names
Country: any = ['England', 'Australia', 'France', 'India']; // dummy list of country to show in the drop down
constructor(public fb: FormBuilder) { }
// a reactive form initialized with a field countryName with no validation.
registrationForm = this.fb.group({
countryName: ['', []]
})
// Country selection
changeCountry(e) {
this.countryName.setValue(e.target.value, {
onlySelf: true
})
}
// Getter method for accessing form controls
get countryName() {
return this.registrationForm.get('countryName');
}
onSubmit() { // method which is called after submit button click
this.isSubmitted = true;
if (!this.registrationForm.valid) {
return false;
} else {
alert(JSON.stringify(this.registrationForm.value))
}
}
}
Step 4: Update template(app.component.html)
<div class="container">
<div class="row custom-wrapper">
<div class="col-md-4 offset-md-4">
<!-- Form starts -->
<form [formGroup]="registrationForm" (ngSubmit)="onSubmit()">
<div class="group-gap">
<div class="d-block my-3">
<div class="mb-3">
<select class="custom-select" (change)="changeCountry($event)" formControlName="countryName">
<option value="">Choose your city</option>
<option *ngFor="let item of Country" [ngValue]="item">{{item}}</option>
</select>
</div>
</div>
</div>
<!-- Submit Button -->
<button type="submit" class="btn btn-primary">Submit</button>
</form><!-- Form ends -->
</div>
</div>
</div>
I have used the bootstrap framework of CSS, Add CDN of bootstrap in index.html file inside the src folder.
Step 5: Run the app
Now run the app with npm start and check the app on browser over URL http://localhost:4200
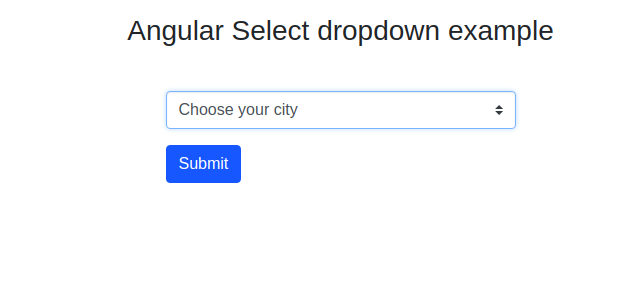
If you want to add validation in Reactive Form. Read my article to get detail about Angular Reactive Form Validation
Conclusion
Working with Form controls in Angular Reactive forms is easy and nowadays Reactive form is more preferred than templated driven form.
Thanks for reading this article. If you are new to Angular then click here find many sample projects to start the app for enterprise-level application
Let me know your thoughts over email demo.jsonworld@gmail.com. I would love to hear them and If you like this article, share it with your friends.