In this tutorial, we'll create an angular application to understand how to build a simple user registration and login system using Angular 8/9, TypeScript and webpack 4.
Below are the requirements for creating the CRUD on MEAN
- Node.js
- Angular CLI
- Angular 8/9
- Mongodb
- IDE or Text Editor
We assume that you have already available the above tools/frameworks and you are familiar with all the above that what individually actually does.
Have a look at the project stucture:
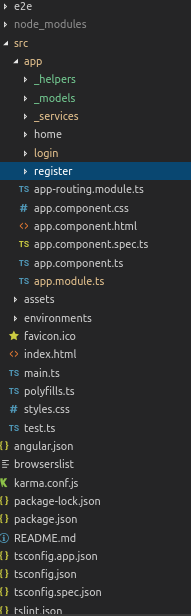
So now we will proceed step by step to achieve the task.
Auth Guard
The auth guard is used to prevent unauthenticated users from accessing restricted routes.
import { Injectable } from '@angular/core';
import { Router, CanActivate, ActivatedRouteSnapshot, RouterStateSnapshot } from '@angular/router';
import { AuthenticationService } from '../_services';
@Injectable({ providedIn: 'root' })
export class AuthGuard implements CanActivate {
constructor(
private router: Router,
private authenticationService: AuthenticationService
) {}
canActivate(route: ActivatedRouteSnapshot, state: RouterStateSnapshot) {
const currentUser = this.authenticationService.currentUserValue;
if (currentUser) {
// authorised so return true
return true;
}
// not logged in so redirect to login page
this.router.navigate(['/login'], { queryParams: { returnUrl: state.url }});
return false;
}
}
User Model
The user model is a class inside the _modals folder that defines the properties of a user form.
export class User {
firstName: string;
lastName: string;
email: string;
phone: number;
password: string;
}
Authentication Service
This is a file under _services folder, It is having functionalities of login and log out of user into the application. The logged in user details are stored in local storage so the user will stay logged in if they refresh the browser and also between browser sessions until they log out.
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { BehaviorSubject, Observable } from 'rxjs';
import { map } from 'rxjs/operators';
import { User } from '../_models';
@Injectable({ providedIn: 'root' })
export class AuthenticationService {
private currentUserSubject: BehaviorSubject<User>;
public currentUser: Observable<User>;
constructor(private http: HttpClient) {
this.currentUserSubject = new BehaviorSubject<User>(JSON.parse(localStorage.getItem('currentUser')));
this.currentUser = this.currentUserSubject.asObservable();
}
public get currentUserValue(): User {
return this.currentUserSubject.value;
}
login(email: string, password: string) {
return this.http.post<any>(`auth/login`, { email, password })
.pipe(map(user => {
if (user && user.token) {
// store user details in local storage to keep user logged in
localStorage.setItem('currentUser', JSON.stringify(user.result));
this.currentUserSubject.next(user);
}
return user;
}));
}
logout() {
// remove user data from local storage for log out
localStorage.removeItem('currentUser');
this.currentUserSubject.next(null);
}
}
User Service
This service is having methods for all the basic user HTTP call like reset password, change password, forgot password request etc.
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { User } from '../_models/user';
@Injectable({ providedIn: 'root' })
export class UserService {
constructor(private http: HttpClient) { }
register(user: User) {
return this.http.post(`auth/register`, user);
}
}
Interceptor Service
Interceptors provide a mechanism to intercept and/or mutate outgoing requests or incoming responses. They are very similar to the concept of middleware with a framework like Express, except for the frontend. Interceptors can be really useful for features like caching and logging
import {
HttpInterceptor,
HttpRequest,
HttpHandler,
HttpEvent
} from "@angular/common/http";
import { Observable } from "rxjs";
import { Injectable } from "@angular/core";
import { environment } from "../../environments/environment";
@Injectable()
export class InterceptorService implements HttpInterceptor {
intercept(
req: HttpRequest<any>,
next: HttpHandler
): Observable<HttpEvent<any>> {
let reqUrl = environment.apiBaseUrl;
req = req.clone({
headers: req.headers.set(
"Authorization",
"Bearer " + localStorage.getItem("token")
),
url: reqUrl +""+ req.url
});
return next.handle(req);
}
}
Home Component Template
Code for basic welcome message
<h1>Hi {{currentUser.firstName}}!</h1>
<p>Welcome to home page!!</p>
Home Component Ts File
This file contains basic class structure for now with currentUser variable which is receiving value from local storage.
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-home',
templateUrl: './home.component.html'
})
export class HomeComponent implements OnInit {
public currentUser;
constructor() {
this.currentUser = localStorage.getItem('currentUser')? JSON.parse(localStorage.getItem('currentUser')) : '';
}
ngOnInit() {
}
}
Login Component Template
A basic form with email and password field with basic validation, And after submit onFormSubmit method will be called inside ts file of the component.
<h3>User Login</h3>
<form [formGroup]="loginForm" (ngSubmit)="onFormSubmit()">
<div class="form-group">
<label for="email">Email</label>
<input type="text" formControlName="email" class="form-control" [ngClass]="{ 'is-invalid': submitted && fval.email.errors }" placeholder="Enter email here"/>
<div *ngIf="submitted && fval.email.errors" class="invalid-feedback">
<div *ngIf="fval.email.errors.required">Email is required</div>
<div *ngIf="fval.email.errors.email">Enter valid email address</div>
</div>
</div>
<div class="form-group">
<label for="password">Password</label>
<input type="password" formControlName="password" class="form-control" [ngClass]="{ 'is-invalid': submitted && fval.password.errors }" placeholder="Enter password here" />
<div *ngIf="submitted && fval.password.errors" class="invalid-feedback">
<div *ngIf="fval.password.errors.required">Password is required</div>
</div>
</div>
<div class="form-group">
<button [disabled]="loading" class="btn btn-primary">
<span *ngIf="loading" class="spinner-border spinner-border-sm mr-1"></span>
Login
</button>
<a routerLink="/register" class="btn btn-link">Register</a>
</div>
</form>
Login Component ts File
File with the code to send login request from this component to authentication service. The toaster has been used to show alerts to the user.
import { Component, OnInit } from '@angular/core';
import { Router, ActivatedRoute } from '@angular/router';
import { FormBuilder, FormGroup, Validators } from '@angular/forms';
import { ToastrService } from 'ngx-toastr';
import { AuthenticationService } from '../_services';
@Component({
selector: 'app-login',
templateUrl: './login.component.html'
})
export class LoginComponent implements OnInit {
loginForm: FormGroup;
loading = false;
submitted = false;
returnUrl: string;
constructor(
private formBuilder: FormBuilder,
private route: ActivatedRoute,
private router: Router,
private authenticationService : AuthenticationService,
private toastr: ToastrService
) { }
ngOnInit() {
this.loginForm = this.formBuilder.group({
email: ['', Validators.required],
password: ['', Validators.required]
});
}
// for accessing to form fields
get fval() { return this.loginForm.controls; }
onFormSubmit() {
this.submitted = true;
if (this.loginForm.invalid) {
return;
}
this.loading = true;
this.authenticationService.login(this.fval.email.value, this.fval.password.value)
.subscribe(
data => {
this.router.navigate(['/']);
},
error => {
this.toastr.error(error.error.message, 'Error');
this.loading = false;
});
}
}
Register Component Template
File with fields of the registration of the new user in the application.
<h3>User Registration</h3>
<form [formGroup]="registerForm" (ngSubmit)="onFormSubmit()">
<div class="form-group">
<label for="firstName">First Name</label>
<input type="text" formControlName="firstName" class="form-control" [ngClass]="{ 'is-invalid': submitted && fval.firstName.errors }" placeholder="Enter First Name here"/>
<div *ngIf="submitted && fval.firstName.errors" class="invalid-feedback">
<div *ngIf="fval.firstName.errors.required">First Name is required</div>
</div>
</div>
<div class="form-group">
<label for="lastName">Last Name</label>
<input type="text" formControlName="lastName" class="form-control" placeholder="Enter Last Name here"/>
</div>
<div class="form-group">
<label for="email">Email</label>
<input type="text" formControlName="email" class="form-control" [ngClass]="{ 'is-invalid': submitted && fval.email.errors }" placeholder="Enter email here"/>
<div *ngIf="submitted && fval.email.errors" class="invalid-feedback">
<div *ngIf="fval.email.errors.required">Email is required</div>
<div *ngIf="fval.email.errors.email">Enter valid email address</div>
</div>
</div>
<div class="form-group">
<label for="email">Phone</label>
<input type="text" formControlName="phone" class="form-control" [ngClass]="{ 'is-invalid': submitted && fval.phone.errors }" placeholder="Enter Phone here"/>
<div *ngIf="submitted && fval.phone.errors" class="invalid-feedback">
<div *ngIf="fval.phone.errors.required">Phone is required</div>
</div>
</div>
<div class="form-group">
<label for="email">Password</label>
<input type="password" formControlName="password" class="form-control" [ngClass]="{ 'is-invalid': submitted && fval.password.errors }" placeholder="Enter Password here"/>
<div *ngIf="submitted && fval.password.errors" class="invalid-feedback">
<div *ngIf="fval.password.errors.required">Password is required</div>
<div *ngIf="fval.password.errors.minlength">Password must be at least 6 characters</div>
</div>
</div>
<div class="form-group">
<button [disabled]="loading" class="btn btn-primary">
<span *ngIf="loading" class="spinner-border spinner-border-sm mr-1"></span>
Register
</button>
<a routerLink="/login" class="btn btn-link">Login to continue</a>
</div>
</form>
Register Component ts File
File with the logical part of the registration process. The registerForm: FormGroup
object defines the form controls and validators and is used to access data entered into the form.
import { Component, OnInit } from '@angular/core';
import { FormBuilder, FormGroup, Validators } from '@angular/forms';
import { Router } from '@angular/router';
import { ToastrService } from 'ngx-toastr';
import { UserService } from '../_services/user.service';
@Component({
selector: 'app-register',
templateUrl: './register.component.html'
})
export class RegisterComponent implements OnInit {
constructor(
private formBuilder: FormBuilder,
private router: Router,
private userService: UserService,
private toastr: ToastrService
) { }
registerForm: FormGroup;
loading = false;
submitted = false;
ngOnInit() {
this.registerForm = this.formBuilder.group({
firstName: ['', Validators.required],
lastName: ['', Validators.required],
phone: ['', Validators.required],
email: ['', [Validators.required,Validators.email]],
password: ['', [Validators.required, Validators.minLength(6)]]
});
}
get fval() { return this.registerForm.controls; }
onFormSubmit(){
this.submitted = true;
// return for here if form is invalid
if (this.registerForm.invalid) {
return;
}
this.loading = true;
this.userService.register(this.registerForm.value).subscribe(
(data)=>{
alert('User Registered successfully!!');
this.router.navigate(['/login']);
},
(error)=>{
this.toastr.error(error.error.message, 'Error');
this.loading = false;
}
)
}
}
App Component Template
The app component template is the root component template of the application, it contains a router-outlet directive for displaying the contents of each view based on the current route.
<!-- main app container -->
<div class="jumbotron">
<a *ngIf="currentUser" class="nav-item nav-link" style="cursor:pointer" (click)="logout()">Logout</a>
<div class="container">
<div class="row">
<div class="col-md-4 offset-md-4">
<router-outlet></router-outlet>
</div>
</div>
</div>
</div>
App Component ts File
The app component is the root component of the application, it defines the root tag of the app as <app></app>
with the selector property of the @Component
decorator.
import { Component } from '@angular/core';
import { Router } from '@angular/router';
import { AuthenticationService } from './_services';
import { User } from './_models';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
currentUser: User;
constructor(
private router: Router,
private authenticationService: AuthenticationService
) {
this.authenticationService.currentUser.subscribe(x => this.currentUser = x);
}
logout() {
this.authenticationService.logout();
this.router.navigate(['/login']);
}
}
App Routing File
Routing for the Angular app is configured as an array of Routes
, each component is mapped to a path so the Angular Router knows which component to display based on the URL in the browser address bar. The home route is secured by passing the AuthGuard to the canActivate property of the route.
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { AuthGuard } from './_helpers/auth.guard';
/**Componenets */
import { LoginComponent } from './login/login.component';
import { RegisterComponent } from './register/register.component';
import { HomeComponent } from './home/home.component';
const routes: Routes = [
{ path: '', component: HomeComponent, canActivate: [AuthGuard] },
{
path: 'login',
component: LoginComponent
},
{
path: 'register',
component: RegisterComponent
},
{ path: '**', redirectTo: '' }
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
App Module
app.module.ts file with all the components, modules, services imported and bootstrapped here in this file. It define the root module of the application along with metadata about the module
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { ReactiveFormsModule } from '@angular/forms';
import { HttpClientModule, HTTP_INTERCEPTORS } from '@angular/common/http';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { ToastrModule } from 'ngx-toastr';
// services
import { InterceptorService } from './_services/interceptor.service';
import { UserService } from './_services/user.service';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { LoginComponent } from './login/login.component';
import { RegisterComponent } from './register/register.component';
import { HomeComponent } from './home/home.component';
@NgModule({
declarations: [
AppComponent,
LoginComponent,
RegisterComponent,
HomeComponent
],
imports: [
BrowserModule,
AppRoutingModule,
ReactiveFormsModule,
HttpClientModule,
BrowserAnimationsModule, // required animations module
ToastrModule.forRoot()
],
providers: [UserService,{ provide: HTTP_INTERCEPTORS, useClass: InterceptorService, multi: true }], //
bootstrap: [AppComponent]
})
export class AppModule { }
Server
Please find the detailed blog on creating server for user registration and login application Start Creating APIs in Node.js with Async/Await
Conclusion
In this demo, we understand the User Registration and login in Angular 8. You can find other demos at Angular sample application
That’s all for now. Thank you for reading and I hope this post will be very helpful for Angular 8 User Registration and Login Example and Tutorial.
Let me know your thoughts over the email demo.jsonworld@gmail.com. I would love to hear them and If you like this article, share with your friends.