In this demo, we will discuss the form validation in angular 8. Since we all come across forms to send data to the server and its needed to validate input from the user. So that desired value can be submitted from the form. Html also provide a basic level of form-validation. Angular also provides is built in form validation. So let's start how form can be validated by angular.
HTML Form with angular validation messages
Let's Get Started
Step 1: Create a new app
So let's move ahead and try to understand it step by step, For this demo, I have just created a basic app of angular 8 by typing ng new template-driven-form-validation.
Step 2: update app.component.html
Open the app.component.html and put the below code in that file.
<div class="container">
<div class="row">
<div class="col-md-4 offset-md-4">
<form name="signup_form" (ngSubmit)="fs.form.valid && signup(fs);" #fs="ngForm" novalidate>
<div class="formbox">
<div class="form-group">
<input type="text" class="form-control" name="fullName" id="fullName" placeholder="Full Name"
name="fullname" [(ngModel)]="user.fullName" minlength="6" #fullName="ngModel" required
pattern="^[A-Za-z ]+$" [ngClass]="{ 'is-invalid': fs.submitted && fullName.invalid }" />
<div *ngIf="fs.submitted && fullName.invalid" class="invalid-feedback">
<div *ngIf="fullName.errors.required">
Full Name is required and must be a valid name
</div>
</div>
</div>
<div class="form-group">
<input type="email" class="form-control" name="email" id="email" placeholder="Email"
[(ngModel)]="user.email" #email="ngModel" pattern="^[a-z0-9._%+-]+@[a-z0-9.-]+.[a-z]{2,4}$"
[ngClass]="{ 'is-invalid': fs.submitted && email.invalid }" required />
<div *ngIf="fs.submitted && email.invalid" class="invalid-feedback">
<div *ngIf="email.errors.required">Email is required</div>
<div *ngIf="email.errors.email">Enter a valid email address</div>
</div>
</div>
<div class="form-group">
<input type="text" class="form-control" name="phone" id="phone" placeholder="Phone No."
[(ngModel)]="user.phone" maxlength="12" minlength="10" #phone="ngModel"
[ngClass]="{ 'is-invalid': fs.submitted && phone.invalid }" required />
<div *ngIf="fs.submitted && phone.invalid" class="invalid-feedback">
<div *ngIf="phone.errors.required">Phone is required</div>
</div>
</div>
<div class="form-group">
<input type="password" class="form-control" name="password" id="password" placeholder="Password"
[(ngModel)]="user.password" #password="ngModel"
[ngClass]="{ 'is-invalid': fs.submitted && password.invalid}" required />
<div *ngIf="fs.submitted && password.invalid" class="invalid-feedback">
<div ngClass="password.errors.required">
Password is required and must be atleast six characters long.
</div>
</div>
</div>
<div class="form-group">
<input type="password" class="form-control" name="confirm_password" id="confirm_password"
placeholder="Confirm Password" [(ngModel)]="confirm_password" #confirmPassword="ngModel"
[ngClass]="{ 'is-invalid': fs.submitted && confirmPassword.invalid }" required
appConfirmEqualValidator='password' />
<div *ngIf="fs.submitted && confirmPassword.invalid" class="invalid-feedback">
<div *ngIf="confirmPassword.errors?.notEqual && !confirmPassword.errors?.required">Password Mismatch</div>
</div>
</div>
<div class="form-group">
<div class="checkboxrow">
<input type="checkbox" id="test1" name="cb" #cb="ngModel" [(ngModel)]="user.cb" [required]="user.cb==null"
[ngClass]="{ 'is-invalid': fs.submitted && cb.invalid }">
<label for="test1" control-label>Agree with terms and conditions</label>
<div *ngIf="fs.submitted && cb.invalid">
<div *ngIf="cb.errors.required">Please agree with terms and conditions</div>
</div>
</div>
</div>
<div class="form-group">
<span style="text-align: center;color: #a94442">{{errorMsg}}</span>
<span style="text-align: center;color: #28a745">{{sucsMsg}}</span>
<button type="submit" class="btn btn-primary signin">Sign Up <span class="arrowbtn"><span
class="fa fa-arrow-right"></span></span></button>
</div>
</div>
</form>
</div>
</div>
</div>
In the above code we have simply used some form fields like name,email, phone, password, confirm password which are available in normal registration process. So I will explain the validations for the above form.
To display error messages a div element is included for every input element like below.
<div *ngIf="fs.submitted && cb.invalid" class="invalid-feedback" >Please agree with terms and conditions</div>
In the above code *ngIf validates the errors if form-field data is not valid. In the above form, we have added fullName, email, phone. And in the error message check with fullName.valid or email.valid or phone.valid.
Step 3: Create a Directive
For matching password and confirm password a custom directive is created in a file named confirm-equal-validator.directive.ts. See the code inside this file.
import { Validator,NG_VALIDATORS, AbstractControl } from '@angular/forms';
import { Directive, Input } from '@angular/core';
@Directive({
selector:'[appConfirmEqualValidator]',
providers: [{
provide: NG_VALIDATORS,
useExisting: ConfirmEqualValidatorDirective,
multi: true
}]
})
export class ConfirmEqualValidatorDirective implements Validator {
@Input() appConfirmEqualValidator: string;
validate(control: AbstractControl):{[key:string]: any} |null {
const controlToCompare = control.parent.get(this.appConfirmEqualValidator);
if(controlToCompare && controlToCompare.value !==control.value){
return { 'notEqual': true}
}
return null;
}
}
Step 4: Update app.component.ts
Created a signup method under this file which will be called on signup form submit. For now, it's having a simple alert for success form submit.
import { Component } from '@angular/core';
declare var NgForm:any;
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor() { }
title = 'template-driven-form-validation-in-angular8';
public user = {};
//this.user.fullName='';
signup(){
alert('form fields are validated successfully!');
}
}
Step 5: Update app.module.ts
Paste the below code inside app.module.ts
import { ConfirmEqualValidatorDirective } from './confirm-equal-validator.directive';
......
.....
declarations: [
AppComponent,ConfirmEqualValidatorDirective
],
Angular 2 and its further version Validation Classes
Angular automatically attached CSS classes to the input elements depending on the state of the control. The following class names are used:
ng-touched
: Control has been visited
ng-untouched
: Control has not been visited
ng-dirty
: Control's value has been changed
ng-pristine
: Control's value hasn't been changed
ng-valid
: Control's value is valid
ng-invalid
: Control's value isn't valid
This way we can implement form validation in angular.
Step 6: Run the app
After completing the needed steps, Run the app with command npm start command over the terminal and check the app over the browser on link http://localhost:4200. After submitting the empty form you will see screen like below:
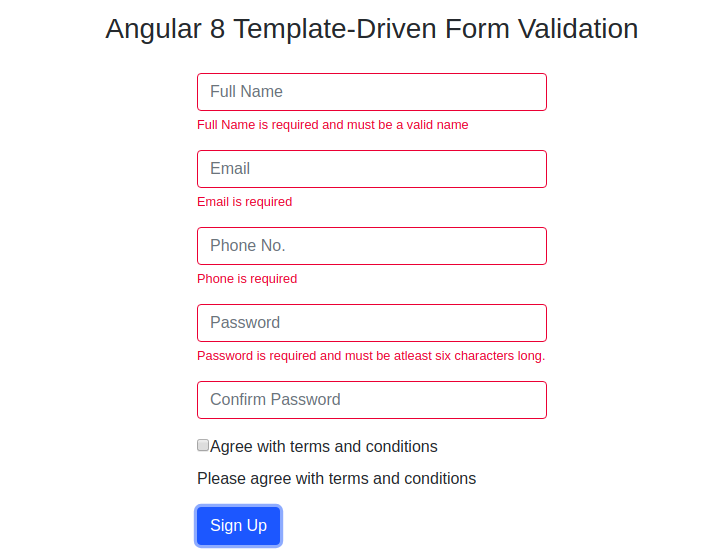
Conclusion
So in this article, I explained how to validate a form with template-driven form validation in Angular 8 application. If you are new to Angular then click here to find many demos to start the app for enterprise-level application
Let me know your thoughts over the email demo.jsonworld@gmail.com. I would love to hear them and If you like this article, share with your friends.
Thanks!!