Today we will create a demo for adress auto complete feature in simple html form using google map api. As a developer we find many places where we need to put this feature where normal user put their adress and we add auto complete option feature to make our application user friendly. Its also very lengthy to take input for complete address, using google map api for address auto complete is also makes the process faster. So if you are working any application having the same feature then this demo can be alot helpful to you. Alot work is not needed here, Its a very simple task only one thing we need to get the API KEY to hit the url provided by google.
Process to get Google API Key
1. Since we hit a url of google to get the suggestions of address on the base of input entered, And for hitting the api url we need google api key. Follow the link below:
https://developers.google.com/maps/documentation/javascript/get-api-key
When link opened in the browser, you will see a button GET A KEY. By clicking on that button you will be directed to a page, Then select create a new project and click Continue button. See the pic below:
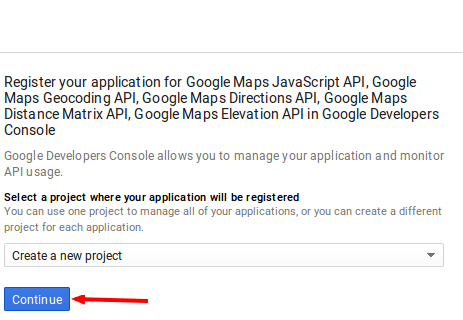
After perform the above steps, Now you’ll able to see your google api management console where you can manage your all google api based projects. here you need to enter your api name by which you can identify your multiple api keys. The page will be like below image:
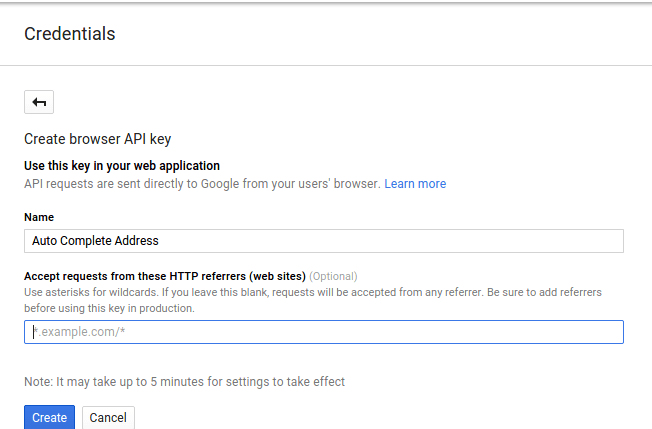
Once you fill the form and click the Create button, A pop up will open with with api key which you can be used for any google api authentication. Now step for generating API KEY is completed and we have a api key which we can use in our api url to get the auto suggestion for address.
CREATE HTML PAGE
Now as I told in the begining that we will create the demo with simple form, So lets have a look on our html code:
<div class=" text-center">
<h3>Address Auto Complete in javascipt using google map api.</h3>
</div>
<div class="row">
<div class="col-sm-6 col-md-offset-3">
<div class="table-responsive">
<table class="table table-striped table-hover table-bordered">
<tr>
<td>Enter address here</td>
<td colspan="2">
<span ><input id="autocomplete" style="width:300px;" placeholder="Enter your address"
onFocus="geolocate()" type="text" /></span>
</td>
</tr>
<tr>
<td>Locality</td>
<td>
<input type="text" id="street_number" disabled="true" />
</td>
<td>
<input type="text" id="route" disabled="true" />
</td>
</tr>
<tr>
<td >City</td>
<td colspan="2"><input id="locality" disabled="true" /></td>
</tr>
<tr>
<td>State</td>
<td colspan="2"><input type="text" id="administrative_area_level_1" disabled="true" /></td>
</tr>
<tr>
<td>Pin Code</td>
<td colspan="2"><input type="text" id="postal_code" disabled="true" /></td>
</tr>
<tr>
<td>Country</td>
<td colspan="2"><input type="text" id="country" disabled="true" /></td>
</tr>
</table>
</div>
</div>
</div>
</div>
As we can see in the above code we have a input field at the top to enter the address and below that we have separate input fields in disabled state to show the adress splited by locality, pincode, state and country.
Now let's have a look in the code in javascript to call the google map api.
var placeSearch, autocomplete;
var componentForm = {
street_number: 'short_name',
route: 'long_name',
locality: 'long_name',
administrative_area_level_1: 'short_name',
country: 'long_name',
postal_code: 'short_name'
};
function initAutocomplete() {
// Create the autocomplete object, restricting the search to geographical
// location types.
autocomplete = new google.maps.places.Autocomplete(
/** @type {!HTMLInputElement} */(document.getElementById('autocomplete')),
{types: ['geocode']});
// When the user selects an address from the dropdown, populate the address
// fields in the form.
autocomplete.addListener('place_changed', fillInAddress);
}
function fillInAddress() {
// Get the place details from the autocomplete object.
var place = autocomplete.getPlace();
for (var component in componentForm) {
document.getElementById(component).value = '';
document.getElementById(component).disabled = false;
}
// Get each component of the address from the place details
// and fill the corresponding field on the form.
for (var i = 0; i < place.address_components.length; i++) {
var addressType = place.address_components[i].types[0];
if (componentForm[addressType]) {
var val = place.address_components[i][componentForm[addressType]];
document.getElementById(addressType).value = val;
}
}
}
// Bias the autocomplete object to the user's geographical location,
// as supplied by the browser's 'navigator.geolocation' object.
function geolocate() {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(function(position) {
var geolocation = {
lat: position.coords.latitude,
lng: position.coords.longitude
};
var circle = new google.maps.Circle({
center: geolocation,
radius: position.coords.accuracy
});
autocomplete.setBounds(circle.getBounds());
});
}
}
In the js code we can see there is a method named geolocate() which is called on the onFocus event, Which is responsible for getting all the suggestions from the google. And rest of the code is used to set the selected address into the different input fields.
That’s all for now . Thank you for reading and I hope this demo(Javascript Sample Application) will be very helpful for adress auto complete option in any web application.
Let me know your thoughts over the email demo.jsonworld@gmail.com. I would love to hear them and If you like this article, share with your friends.